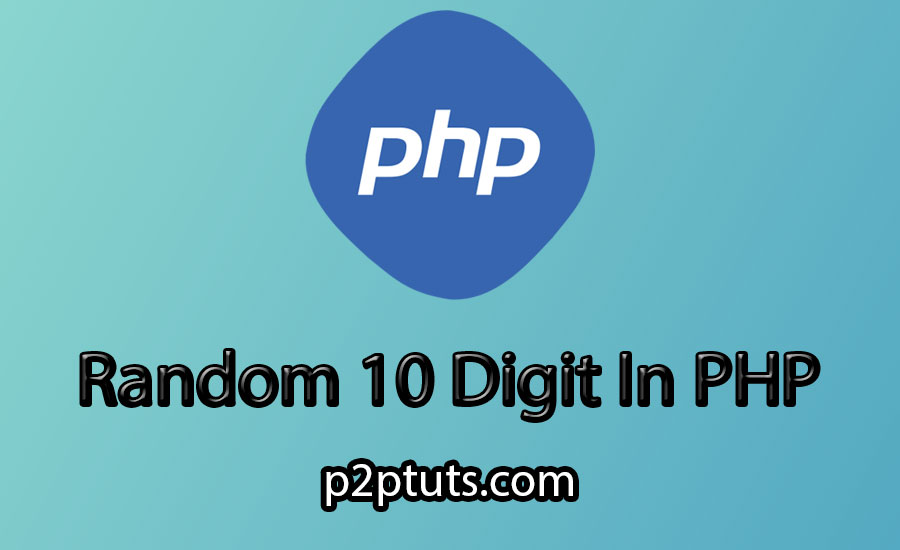
How To Create 10 Digit Random Number Generator In PHP
In many PHP applications, there is a need to generate random numbers, especially those with 10 digits, for situations such as creating mock phone numbers, verification codes, or other purposes. Below is how you can 10 digit random number generator in php this using PHP.
Using the rand() Function
The rand()
function in PHP is an efficient tool for generating random numbers within a specified range. To create a random number with 10 digits, you can use rand()
in combination with a simple calculation. Here is an example source code:
<?php
function generateRandom10DigitNumber() {
$min = 1000000000; // Minimum value (10 digits)
$max = 9999999999; // Maximum value (10 digits)
$randomNumber = rand($min, $max);
return $randomNumber;
}
// Call the function to get a random number
$random10DigitNumber = generateRandom10DigitNumber();
// Display the result
echo "Random 10-digit number: " . $random10DigitNumber;
?>
In the example above, the generateRandom10DigitNumber()
function uses rand()
to generate a random number within the range from 10 billion to 99 billion 999 million 999 thousand 999 (the range for a 10-digit number). The random 10 digit number is then returned and displayed.
Using the mt_rand() Function
Below is a PHP code snippet using the mt_rand() function to generate a random 10-digit integer:
<?php
function generate_random_number() {
return mt_rand(1000000000, 9999999999);
}
echo "Random number generated using mt_rand() function is " . generate_random_number();
?>
In the above code snippet, we utilize the mt_rand() function to generate a random 10-digit integer within the range from 10^9 to 10^10 - 1. Finally, we use the echo() function to display the randomly generated number.
Note: For very large numbers, you may encounter issues with the limitations of the integer data type in PHP. In such cases, you may consider using the BCMath library to handle larger numbers.
Summary
Both rand()
and mt_rand()
functions provide the functionality to generate random numbers in PHP. However, mt_rand()
offers better random number quality and is often preferred. Hopefully, through this article, you have gained a better understanding of how to use these two functions in PHP programming.