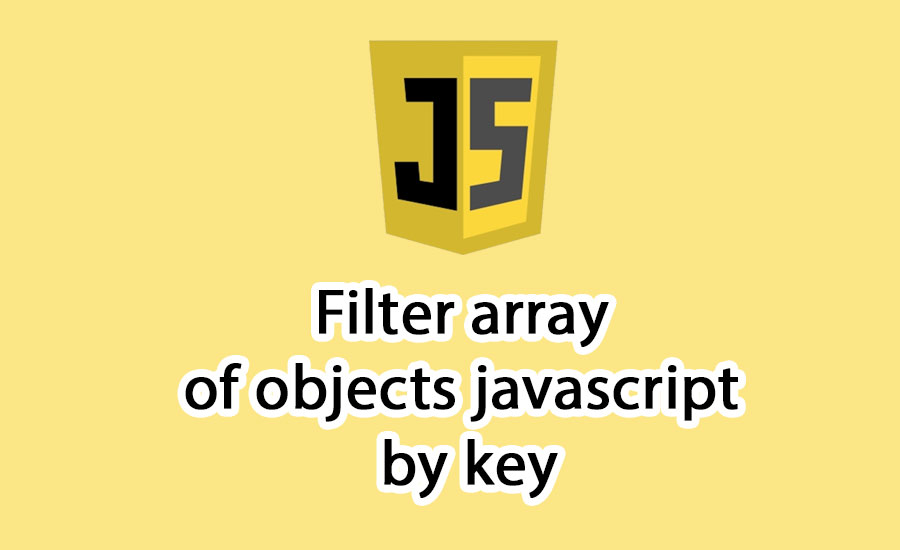
How to filter array of objects javascript by key
- by Wilson
- 101 Views
- NodeJS
- Javascript
Filtering arrays of objects is a common task in JavaScript when you need to extract specific data based on certain criteria. One powerful technique is filtering by key, where you extract objects that match a particular property. Let’s delve into how you can achieve this effortlessly.
Understanding the Concept
Imagine you have an array of language objects, each containing information about a specific programming language. You might want to filter this array to extract only those languages with certain characteristics, such as popularity or age.
The Process
We can accomplish this task using the filter()
method in JavaScript, which creates a new array with all elements that pass the test implemented by the provided function. Here's how you can do it:
// Sample array of language objects
let languages = [
{ name: 'JavaScript', popularity: 'High' },
{ name: 'Python', popularity: 'High' },
{ name: 'Ruby', popularity: 'Medium' },
{ name: 'Go', popularity: 'Medium' }
];
// Function to filter array of objects by key
function filterByKey(arr, key, value) {
return arr.filter(item => item[key] === value);
}
// Example: Filtering languages by popularity
let popularLanguages = filterByKey(languages, 'popularity', 'High');
console.log('Popular languages:', popularLanguages);
In this example, we have an array of language objects with properties like name
and popularity
. The filterByKey
function takes three parameters: the array to filter, the key to filter by, and the desired value. It then returns a new array containing only the objects where the specified key matches the given value.
Conclusion
How to filter array of objects javascript by key. By leveraging the filter()
method and a custom filtering function, you can efficiently extract data based on specific criteria, streamlining your code and improving readability.