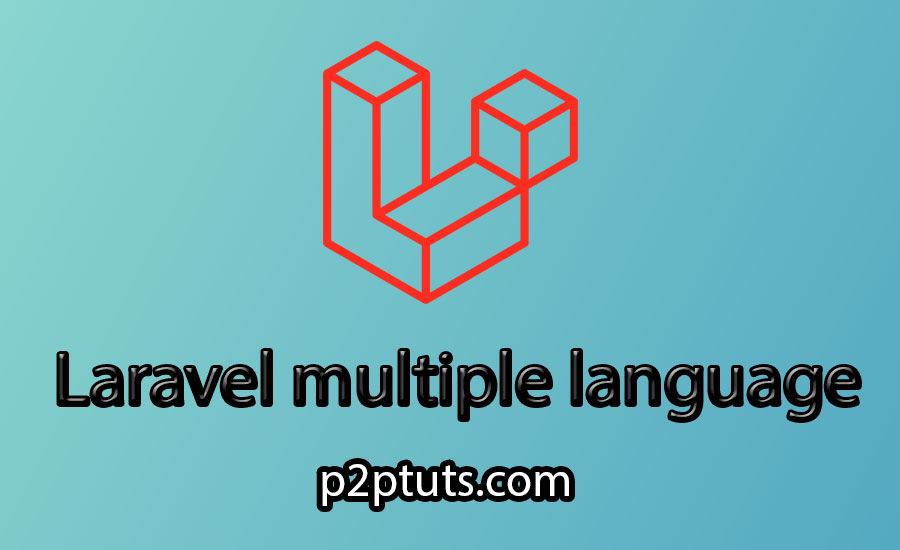
Laravel Multi Language: How to Translate Your Application Effectively
Currently, websites are targeting users in various regions worldwide, so it is necessary to use laravel multi languages on the website. With Laravel, you can easily implement multilingual support for your website. This article will guide you on how to use multiple languages in Laravel
Installing Language Files
In Laravel version 8 and below, the default language folder is located in resources/lang
. For version 9, the language folder is outside the root, and for Laravel 10, the language folder is hidden. To use it, run the following command:
php artisan lang:publish
This command will generate a lang
folder parallel to the root.
Laravel supports two language formats: .php
or .json
, placed in language-specific folders, as shown below:
- For
.php
format:
/lang
/en
messages.php
/es
messages.php
- For
.json
format:
/lang
en.json
es.json
Configuring Language File Content
For .php
files, you can use an array format like this:
<?php
// lang/en/messages.php
return [
'welcome' => 'Welcome to Website',
'welcome_name' => 'Welcome, :name',
];
Looking at the code above, you can see how to pass content based on the key, :name is the input parameter if you want details about a certain object.
For .json
files, the structure is similar to PHP:
{
'welcome' => 'Welcome to Website!',
'welcome_name' => 'Welcome, :name'
}
Using in Blade Views
To use in Blade views, you can use the following syntax:
echo __('messages.welcome');
//or
{{ __('messages.welcome') }}
Note: For JSON files, you don't need to include 'messages'.
Setting Default Language
Configure the language in config/app.php
by editing the following parameters:
'locale' => 'en',
// Note: 'en' is the folder name in lang/en or the name of the en.json file
and a fallback language
'fallback_locale' => 'en',
Using Session and Middleware
To allow users to customize their language, use sessions and middleware:
Example:
public function setLang($language)
{
\Session::put('language', $language);
return redirect()->back();
}
First, I will create a path into the setLang function and I will create a session name language
public function handle($request, Closure $next)
{
$language = \Session::get('language', config('app.locale'));
config(['app.locale' => $language]);
return $next($request);
}
protected $routeMiddleware = [
'locale' => \App\Http\Middleware\Locale::class,
];
Go to app/Http/Kernel.php then add middleware in this file and in web.php you can easily use it.
Conclusion
In summary, p2ptips.com has provided a guide on how to use multiple languages in Laravel. We hope it proves useful for your application.