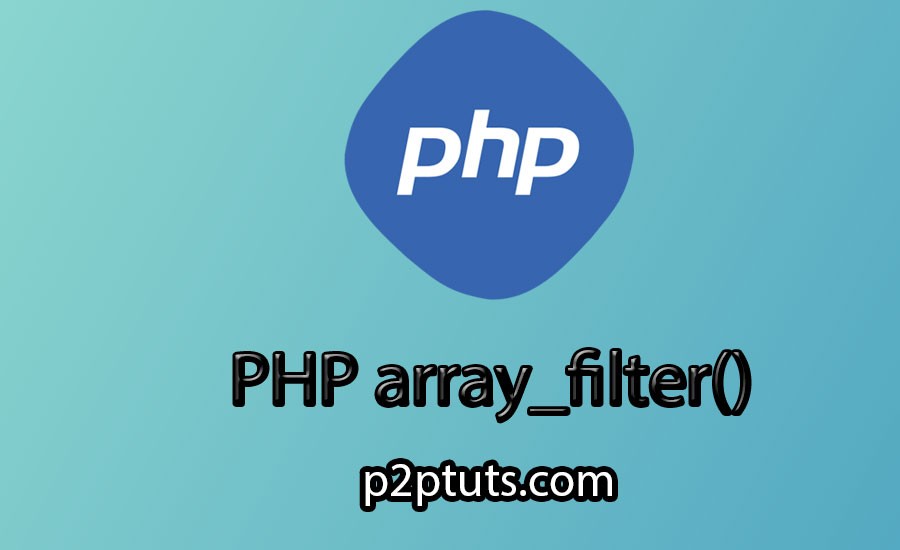
How to use array_filter() function in php
PHP, known for its strength and flexibility, provides developers with a variety of built-in functions for working with arrays. Among these functions, array_filter
stands out as a useful tool, allowing the filtering of array elements based on specified conditions. Let's delve into the details of this function and its usage in php array filter.
The array_filter Function in PHP
Basic Syntax
array_filter(array $array, ?callable $callback = null, int $mode = 0): array
$array
: The input array to be filtered.$callback
: (Optional) The callback function that determines which elements to include in the result. If not provided, all elements of the array are included in the result.$flag
: (Optional) A flag determining what arguments are sent to the callback function. Default is 0.
Purpose of Usage
The array_filter
function helps filter array elements based on specific conditions, creating a new array containing only elements that satisfy those conditions. This proves helpful when cleaning data or focusing on a specific part of the array.
Practical Example
Let's consider an array containing students' grades:
$studentGrades = [7.5, 8.2, 6.9, 9.5, 5.8, 7.0];
Suppose we want to filter out the grades of students with scores greater than or equal to 8. Using array_filter
and a callback function:
function filterExcellentStudents($grade) {
return $grade >= 8;
}
$excellentStudents = array_filter($studentGrades, 'filterExcellentStudents');
The result will be a new array containing the grades of students who performed exceptionally well. This facilitates the creation of a new dataset based on custom criteria.
Summary
The array_filter
function in PHP is a powerful tool for easy and effective array processing. Its usage reduces the need for iterating through arrays and checking conditions manually, instead creating a new array containing only the essential elements. This enhances flexibility and simplifies data processing in PHP applications.